Web Push
Push messaging provides a simple and effective way to re-engage with your users. Once users subscribed, vendor could push messaging for them.
<msc-web-push />
provides a simple usage for developers. It will handle subscription process and UI mutations.
Tap the following button and see what can <msc-web-push />
do.
Push messageing test
Developers could use the following ways to test push messageing after users subscribed.
Chrome DevTools
Turn on DevTools and switch panel to Application
. Input test data in Push input field then press Push
button. ( )
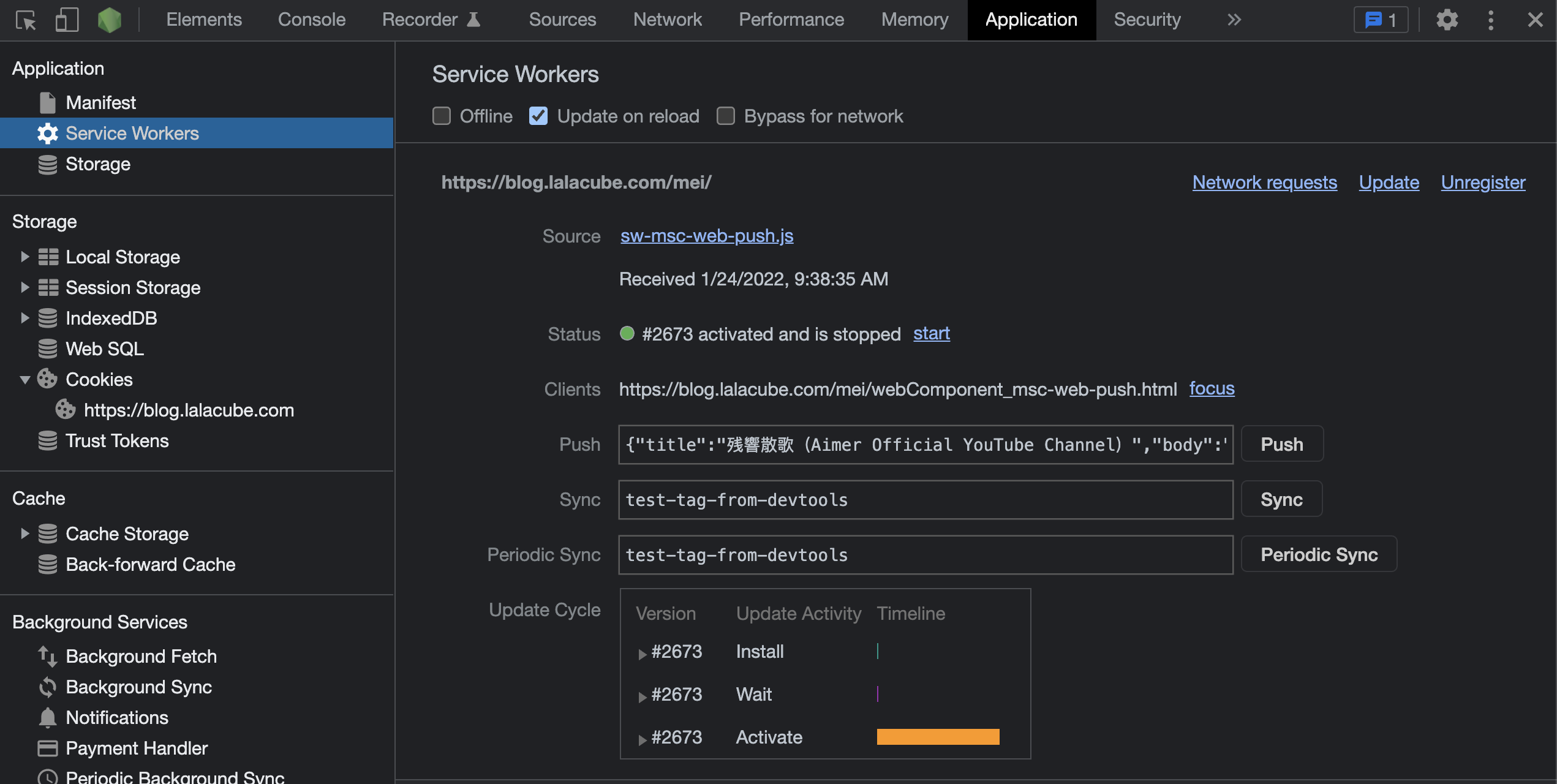
Web Push Code Lab
Visit Web Push Code Lab. Developers could type Subscription
& Message
in <textarea /> then press SEND PUSH MESSAGE
button.
Basic Usage
<msc-web-push />
is a web component. All we need to do is put the required script into your HTML document. Then follow <msc-web-push />
's html structure and everything will be all set.
Required Script
Structure
Put clickable content inside <msc-web-push />
as its child and set attribute "slot" as "msc-web-push-trigger".
It will have subscribe / unsubscribe feature when user tapped.
PS. Both of "service-worker-path
" & "public-key
" must be set. Developers could generate public / private key through Web Push CodeLab.
Set config through attribute is acceptable. Above structure could change as following code.
Otherwise, developers could also choose remoteconfig to fetch config for <msc-web-push />.
JavaScript Instantiation
<msc-web-push />
could also use JavaScript to create DOM element. Here comes some examples.
Style Customization
<msc-web-push />
will add attribute "subscribed
" when user subscribed. That means developers could use the follwoing selector to style the clickable element.
Attributes
<msc-web-push />
supports some attributes to let it become more convenience & useful.
service-worker-path
Set service-worker-path for <msc-web-push />
.
public-key
Set public-key for <msc-web-push />
.
Properties
Property Name | Type | Description |
---|---|---|
service-worker-path
|
String | Getter / Setter for service-worker-path. |
public-key
|
String | Getter / Setter for public-key. |
subscribed
|
Boolean | Getter for subscription status. |
Method
Method Signature | Description |
---|---|
getSubscription
|
Get current subscription data. |
Event
Event Signature | Description |
---|---|
msc-web-push-subscription-change
|
Fired when <msc-web-push /> subscription changed. |